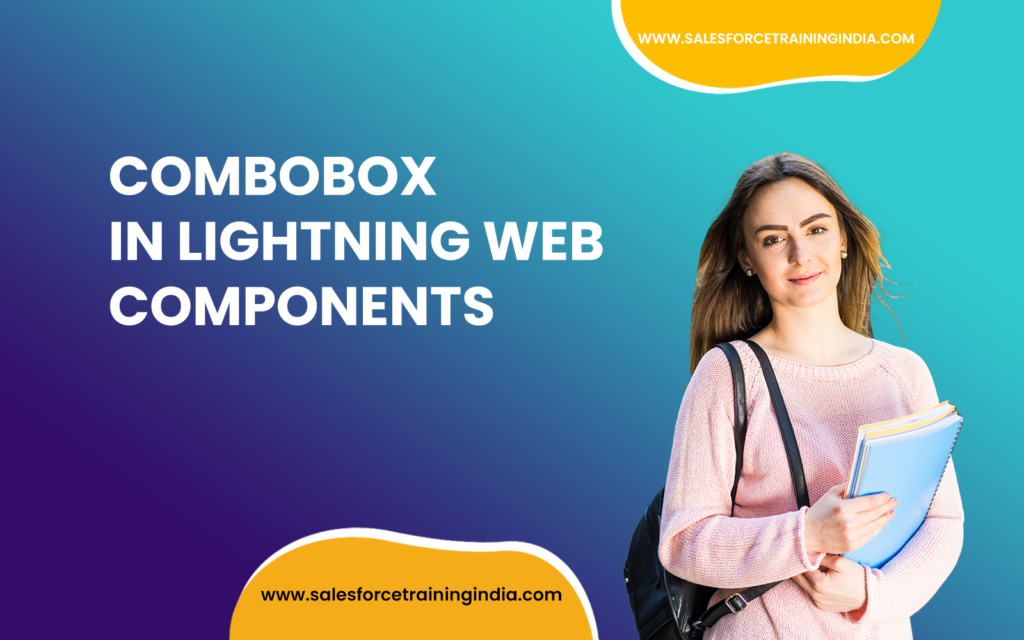
Definition of Combobox LWC
A combobox in Lightning Web Components (LWC) is a powerful UI component that allows users to select an option from a predefined list of choices. This component is a combination of a dropdown list and an editable text box, providing both the convenience of a dropdown and the flexibility of a text input field. The combobox is particularly useful in scenarios where users need to either select from existing options or input their own custom value.
Comboboxes in LWC enhance user experience by providing an intuitive way to select and search for items. They can be used in various forms and applications, making data entry and selection processes more efficient and user-friendly. By leveraging the combobox component, developers can create dynamic and responsive user interfaces that cater to a wide range of user inputs and preferences.
Steps to Create a New LWC Component
Creating a new LWC component involves a series of straightforward steps. Here’s a step-by-step guide to help you get started:
- Set Up Your Salesforce DX Environment: Before creating an LWC component, ensure that your Salesforce DX environment is set up. This includes installing Salesforce CLI and creating or connecting to a Salesforce DX project.
- Create a New LWC Component: Open your terminal or command prompt and navigate to your Salesforce DX project directory. Use the following command to create a new LWC component:
sfdx force:lightning:component:create –type lwc –componentname comboboxComponent –outputdir force-app/main/default/lwc
This command will generate the necessary files for your LWC component in the specified directory.
Edit the Component Files: Navigate to the comboboxComponent
folder within your project directory. You will find three main files: comboboxComponent.html
, comboboxComponent.js
, and comboboxComponent.js-meta.xml
. Edit these files to define the structure, functionality, and metadata of your combobox component.
Implement the Combobox Logic: In the comboboxComponent.js
file, implement the logic for the combobox. This includes defining the options for the combobox and handling user input and selection.
Deploy the Component to Salesforce: Once your component is ready, deploy it to your Salesforce org using the following command:
sfdx force:source:deploy -p force-app
This will make your new LWC component available in your Salesforce org.
File Structure of an LWC Component
Understanding the file structure of an LWC component is crucial for efficient development. Here’s a breakdown of the typical file structure for an LWC component:
HTML File (comboboxComponent.html
): This file contains the template for your LWC component. It defines the structure and layout of the component’s UI. For a combobox, this might include the markup for the dropdown list and input field.
<template>
<lightning-combobox
name="combobox"
label="Select an Option"
value={value}
placeholder="Choose an option"
options={options}
onchange={handleChange}>
</lightning-combobox>
</template>
JavaScript File (comboboxComponent.js
): This file contains the component’s logic and functionality. It defines properties, methods, and event handlers that drive the behavior of the component.
import { LightningElement, track } from 'lwc';
export default class ComboboxComponent extends LightningElement {
@track value = '';
@track options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' },
];
handleChange(event) {
this.value = event.detail.value;
}
}
Metadata Configuration File (comboboxComponent.js-meta.xml
): This XML file provides metadata about the LWC component, such as its API version and visibility. It controls how the component is exposed within the Salesforce environment.
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata" fqn="comboboxComponent">
<apiVersion>55.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>
Attributes of the <lightning-combobox>
Tag
The <lightning-combobox>
tag is a versatile component in LWC, designed to create a combination of a dropdown list and an editable text box. Understanding its attributes is essential for effectively utilizing this component. Here are the key attributes of the <lightning-combobox>
tag:
name: This attribute specifies the name of the combobox component. It is useful for identifying the component when handling events or in form submissions.
<lightning-combobox name="myCombobox" ... ></lightning-combobox>
label: This attribute provides a label for the combobox, which is displayed to the user. It helps in identifying the purpose of the combobox.
<lightning-combobox label="Select an Option" ... ></lightning-combobox>
value: This attribute binds the selected value of the combobox. It is a two-way binding, meaning it reflects the currently selected option and can be updated programmatically.
<lightning-combobox value={selectedValue} ... ></lightning-combobox>
placeholder: This attribute specifies a placeholder text that appears when no option is selected. It provides a hint to the user about the expected input.
<lightning-combobox placeholder="Choose an option" ... ></lightning-combobox>
JavaScript for Handling Combobox
To handle the combobox in a Lightning Web Component, you need to define the necessary properties and methods in the JavaScript file. This includes managing the selected value and handling user input events. Here’s an example:
import { LightningElement, track } from 'lwc';
export default class ComboboxComponent extends LightningElement {
@track selectedValue = '';
@track options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' },
];
handleChange(event) {
this.selectedValue = event.detail.value;
}
}
In this example, selectedValue
is a tracked property that holds the currently selected value from the combobox. The options
array contains the list of options that will be displayed in the dropdown. The handleChange
method is an event handler that updates selectedValue
when the user selects a different option.
How to Create a Custom Dropdown in the Lightning Web Component
Creating a custom dropdown in LWC involves building the dropdown UI and implementing the necessary logic to manage its behavior. Here’s a simple example:
- HTML File (
customDropdown.html
):
<template>
<div class="dropdown">
<button class="dropdown-button" onclick={toggleDropdown}>{selectedLabel}</button>
<div class="dropdown-content" if:true={isDropdownOpen}>
<template for:each={options} for:item="option">
<div key={option.value} class="dropdown-item" onclick={selectOption} data-value={option.value}>
{option.label}
</div>
</template>
</div>
</div>
</template>
JavaScript File (customDropdown.js
):
import { LightningElement, track } from 'lwc';
export default class CustomDropdown extends LightningElement {
@track isDropdownOpen = false;
@track selectedLabel = 'Select an option';
@track options = [
{ label: 'Option 1', value: 'option1' },
{ label: 'Option 2', value: 'option2' },
{ label: 'Option 3', value: 'option3' },
];
toggleDropdown() {
this.isDropdownOpen = !this.isDropdownOpen;
}
selectOption(event) {
const value = event.currentTarget.dataset.value;
const selectedOption = this.options.find(option => option.value === value);
this.selectedLabel = selectedOption.label;
this.isDropdownOpen = false;
}
}
CSS File (customDropdown.css
):
.dropdown {
position: relative;
display: inline-block;
}
.dropdown-button {
background-color: #f9f9f9;
border: 1px solid #ccc;
padding: 10px;
cursor: pointer;
}
.dropdown-content {
display: block;
position: absolute;
background-color: #f9f9f9;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
}
.dropdown-item {
padding: 12px 16px;
cursor: pointer;
}
.dropdown-item:hover {
background-color: #f1f1f1;
}
Deploy and Use the Component
To deploy and use your LWC component, follow these steps:
Deploy the Component: Ensure you have saved all your component files (customDropdown.html
, customDropdown.js
, and customDropdown.css
) in the correct directory within your Salesforce project. Use the following command to deploy your component to your Salesforce org:
sfdx force:source:deploy -p force-app/main/default/lwc/customDropdown
Use the Component: Once deployed, you can use your custom dropdown component in a Salesforce Lightning page. Here’s how you can include the component in a Lightning App or another LWC component:
In a Lightning App:
<template>
<c-custom-dropdown></c-custom-dropdown>
</template>
In Another LWC Component:
<template>
<c-custom-dropdown></c-custom-dropdown>
</template>
After deploying and including your component, navigate to the appropriate page in your Salesforce org to see your custom dropdown in action.
By following these steps, you can effectively create, deploy, and use a combobox or custom dropdown in Lightning Web Components, enhancing the interactivity and usability of your Salesforce applications.
Frequently Asked Questions (FAQs)
1. What is a combobox in Lightning Web Components (LWC)?
A combobox in Lightning Web Components (LWC) is a user interface element that allows users to select an option from a dropdown list. It combines the features of a text input field and a dropdown list, providing a convenient way to present a list of selectable options. The lightning-combobox
component in LWC is used to implement this functionality, offering a native look and feel consistent with the Salesforce Lightning Design System.
2. How do you create a combobox in LWC?
To create a combobox in LWC, you use the lightning-combobox
component. Define the component in your HTML template file with required attributes such as name
, label
, and options
. The options
attribute is an array of objects, each containing a label
and a value
. This array populates the dropdown list. In your JavaScript controller, you can initialize and manage the options array. The value
attribute can be used to bind the selected value of the combobox to a property in your component.
3. What are the key properties of the lightning-combobox component?
The key properties of the lightning-combobox
component include:
- label: The text label for the combobox.
- options: An array of objects defining the selectable options, each with
label
andvalue
properties. - value: The currently selected value.
- placeholder: Text displayed when no option is selected.
- name: A unique identifier for the combobox.
- disabled: Boolean indicating whether the combobox is disabled. These properties allow for flexible configuration and integration with your LWC component.
4. How can you populate a combobox with dynamic data in LWC?
To populate a combobox with dynamic data in LWC, you fetch the data from an external source, such as a Salesforce Apex controller or a REST API, and then format it into an array of objects with label
and value
properties. Assign this array to the options
attribute of the lightning-combobox
component. You can use lifecycle hooks like connectedCallback
to initialize the data when the component is loaded. This approach ensures that the combobox options are dynamically populated based on real-time data.
5. Can you customize the appearance of a combobox in LWC?
While the lightning-combobox component is designed to follow the Salesforce Lightning Design System, its appearance can be customized to some extent using custom CSS. You can apply styles to the combobox by defining CSS classes in the component’s CSS file and using the class
attribute in the lightning-combobox
tag. However, extensive customization might be limited, as the component is intended to maintain a consistent look and feel across the Salesforce platform. For more advanced styling, consider creating a custom combobox component using standard HTML and CSS combined with LWC capabilities.
6. How do you handle events triggered by a combobox selection in LWC?
To handle events triggered by a combobox selection in LWC, you use the onchange
attribute of the lightning-combobox
component. This attribute specifies a handler function in your JavaScript controller that will be called whenever the selected value changes. Within this handler function, you can access the selected value using the event.target.value
property. This allows you to perform any necessary actions based on the user’s selection, such as updating other parts of the UI, making server calls, or processing data.
7. What are the limitations of using a combobox in LWC?
While the lightning-combobox component is powerful and flexible, it has some limitations. One key limitation is the lack of support for multi-select functionality; the component only allows single value selection. Another limitation is the customization constraint, as the component is designed to adhere to the Salesforce Lightning Design System, limiting extensive styling changes. Additionally, handling a very large list of options might impact performance, requiring developers to implement pagination or search functionality for better user experience.
8. How do you preselect a value in a combobox in LWC?
To preselect a value in a combobox in LWC, you set the value
attribute of the lightning-combobox
component to the desired value. This should match one of the values in the options array. You can initialize this value in your JavaScript controller, either through hardcoded values, from data fetched from a server, or based on certain conditions. By setting the value
attribute appropriately, the combobox will display the preselected option when the component is rendered.
9. Can you use a combobox inside a Lightning Datatable in LWC?
Yes, you can use a combobox inside a Lightning Datatable in LWC by defining custom data types for your datatable columns. This involves creating a custom cell renderer component that includes the lightning-combobox
component. You then specify this custom renderer in the columns
attribute of your lightning-datatable
component. This approach allows you to provide dropdown selections for specific cells within the datatable, enabling dynamic and interactive data manipulation directly within the table.
10. How do you validate the selection of a combobox in LWC?
To validate the selection of a combobox in LWC, you can implement validation logic in the onchange
handler or a separate validation function. This logic can check if the selected value meets certain criteria, such as being non-empty or matching a specific format. If the selection is invalid, you can display an error message using the setCustomValidity
and reportValidity
methods on the combobox element. This ensures that users are informed about any validation issues and can correct their selections accordingly, enhancing the overall user experience.