In Lightning Web Components (LWC), buttons are a fundamental UI element used to trigger actions and handle user interactions. The lightning-button
component in LWC offers a versatile and customizable way to implement buttons in your Salesforce applications. This guide will walk you through the usage, customization, and best practices for lightning-button
in LWC.
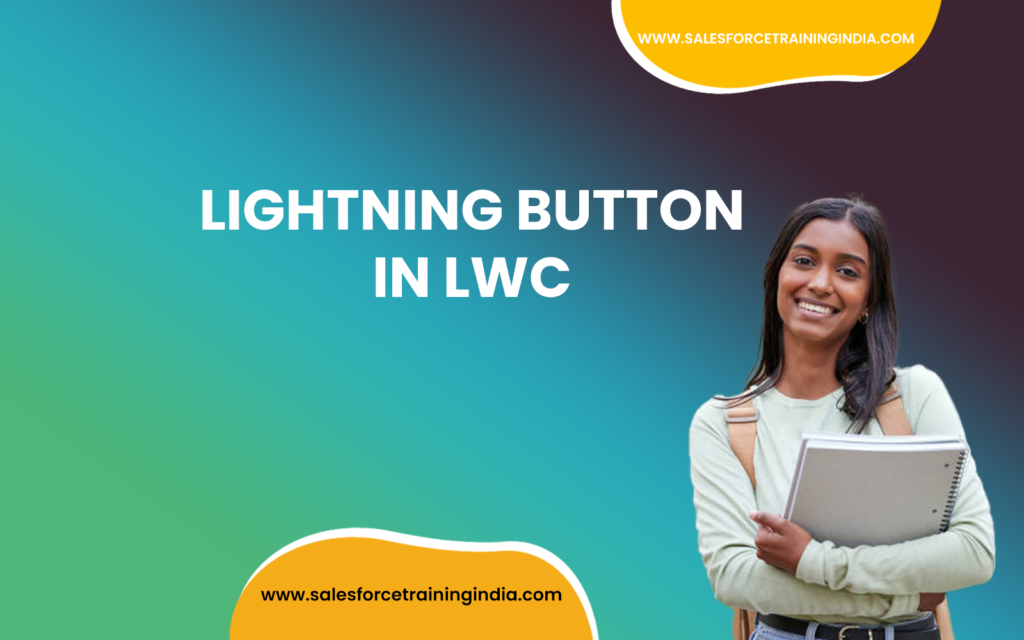
Basic Usage
To use a lightning-button
in an LWC component, you need to include it in your component’s HTML file. Here is a simple example:
<template>
<lightning-button label="Click Me" onclick={handleClick}></lightning-button>
</template>
In this example, a button with the label “Click Me” is created, and it triggers the handleClick
function when clicked.
Handling Button Clicks
To handle the button click event, you define the handleClick
method in your component’s JavaScript file:
import { LightningElement } from 'lwc';
export default class ButtonExample extends LightningElement {
handleClick() {
// Logic to execute when the button is clicked
console.log('Button was clicked!');
}
}
Customizing Button Appearance
The lightning-button
component provides several attributes to customize its appearance:
- label: Specifies the text displayed on the button.
- variant: Defines the button style. Common values are
brand
,neutral
,destructive
, andsuccess
. - icon-name: Adds an icon to the button. You can use any standard Salesforce icon.
- disabled: Disables the button if set to
true
.
Here is an example with various customizations:
<template>
<lightning-button label="Primary Button" variant="brand" onclick={handleClick}></lightning-button>
<lightning-button label="Neutral Button" variant="neutral" onclick={handleClick}></lightning-button>
<lightning-button label="Success Button" variant="success" onclick={handleClick}></lightning-button>
<lightning-button label="Destructive Button" variant="destructive" onclick={handleClick}></lightning-button>
<lightning-button label="Icon Button" icon-name="utility:download" onclick={handleClick}></lightning-button>
<lightning-button label="Disabled Button" disabled="true"></lightning-button>
</template>
Example of a Functional Component
Here is a complete example of an LWC component that uses lightning-button
with different variants and a click handler:
buttonExample.html
<template>
<lightning-card title="Button Example">
<lightning-button label="Primary" variant="brand" onclick={handleClick}></lightning-button>
<lightning-button label="Neutral" variant="neutral" onclick={handleClick}></lightning-button>
<lightning-button label="Success" variant="success" onclick={handleClick}></lightning-button>
<lightning-button label="Destructive" variant="destructive" onclick={handleClick}></lightning-button>
<lightning-button label="Icon Button" icon-name="utility:download" onclick={handleClick}></lightning-button>
<lightning-button label="Disabled" disabled="true"></lightning-button>
</lightning-card>
</template>
buttonExample.js
import { LightningElement } from 'lwc';
export default class ButtonExample extends LightningElement {
handleClick(event) {
const label = event.target.label;
console.log(`${label} button was clicked!`);
}
}
Frequently Asked Questions (FAQs)
1. What is the lightning action button in LWC?
A lightning action button in LWC (Lightning Web Components) is a button component that users can add to their Salesforce Lightning pages or components to trigger specific actions. These buttons are built using the Lightning Design System, which ensures they adhere to Salesforce’s design guidelines, providing a consistent user experience. The button can execute JavaScript code to perform tasks such as navigating to a different page, calling an Apex method, or invoking another LWC component. The lightning-button
base component is commonly used to create these buttons in LWC, offering various styles and behaviors out of the box.
2. How do I create a lightning button in Salesforce?
To create a lightning button in Salesforce using LWC, follow these steps:
- Create a Lightning Web Component: First, create a new LWC. In the Salesforce CLI, run the command:
fdx force:lightning:component:create --type lwc --componentname myButtonComponent --outputdir force-app/main/default/lwc
- Define the Button in HTML: In the
myButtonComponent.html
file, use thelightning-button
component to create the button:<template> <lightning-button label="Click Me" onclick={handleClick}></lightning-button> </template>
- Add JavaScript Logic: In the
myButtonComponent.js
file, define thehandleClick
method to specify what happens when the button is clicked:import { LightningElement } from 'lwc'; export default class MyButtonComponent extends LightningElement { handleClick() { // Add your custom logic here console.log('Button clicked!'); } }
- Deploy the Component: Deploy the component to your Salesforce org:
fdx force:source:deploy -p force-app
- Add the Component to a Lightning Page: Finally, add the LWC to a Lightning page using the Lightning App Builder.
3. How do I add a LWC component to a button?
To add a LWC component to a button, you typically use an event handler to render or navigate to the component. Here’s how:
- Create the LWC Component: Assume you have a component named
myNewComponent
. - Define the Button in the Parent Component: In your parent component’s HTML file, include the
lightning-button
:<template> <lightning-button label="Show Component" onclick={showComponent}></lightning-button> <template if:true={isComponentVisible}> <c-my-new-component></c-my-new-component> </template> </template>
- Handle the Button Click in JavaScript: In the parent component’s JS file, control the visibility of the new component:
import { LightningElement, track } from 'lwc'; export default class ParentComponent extends LightningElement { @track isComponentVisible = false; showComponent() { this.isComponentVisible = true; } }
4. How to change background color of lightning button in LWC?
To change the background color of a lightning button in LWC, you can use custom CSS. Here’s an example:
- Create or Update the Component’s CSS File: Add or update the CSS file (e.g.,
myButtonComponent.css
):.customButton { background-color: #FF5733; /* Your custom color */ color: white; border: none; }
- Apply the Custom CSS Class: In the component’s HTML file, apply the custom CSS class to the
lightning-button
:<template> <lightning-button label="Custom Color" class="customButton" onclick={handleClick}></lightning-button> </template>
5. Why is lightning element used in LWC?
The lightning
elements in LWC are part of the Lightning Design System, which provides pre-built components that ensure a consistent look and feel across Salesforce applications. These components are designed to be reusable, accessible, and compatible with Salesforce’s design principles. Using lightning
elements offers several benefits:
- Consistency: Ensures a unified user experience that aligns with Salesforce’s UI/UX standards.
- Productivity: Speeds up development by providing ready-to-use, well-tested components.
- Accessibility: Built-in accessibility features help make your application usable by everyone, including those with disabilities.
- Maintainability: Standard components are regularly updated by Salesforce, ensuring they stay current with design trends and best practices.
Incorporating lightning elements in LWC helps developers build robust, visually appealing, and user-friendly applications more efficiently.
6. How do you give space between two lightning buttons in LWC?
To give space between two lightning buttons in LWC, you can use CSS to add margin to the buttons. Here’s an example:
- Create or Update the Component’s CSS File: Add margin to the button class in the CSS file (e.g.,
myButtonComponent.css
):.buttonSpacing { margin-right: 10px; /* Adjust the value as needed */ }
- Apply the Custom CSS Class: In the component’s HTML file, apply the custom CSS class to the
lightning-button
elements:<template> <lightning-button label="Button 1" class="buttonSpacing" onclick={handleClick}></lightning-button> <lightning-button label="Button 2" onclick={handleClick}></lightning-button> </template>
7. How to align a button in LWC?
To align a button in LWC, you can use CSS flexbox for different types of alignment:
- Horizontal Alignment: To center align a button horizontally:
<template> <div class="buttonContainer"> <lightning-button label="Center Button" onclick={handleClick}></lightning-button> </div> </template>
.buttonContainer { display: flex; justify-content: center; }
- Vertical Alignment: To center align a button vertically within a container:
<template> <div class="buttonContainer"> <lightning-button label="Center Button" onclick={handleClick}></lightning-button> </div> </template>
.buttonContainer { display: flex; align-items: center; height: 100vh; /* Full viewport height */ }
8. How do I enable lightning in Salesforce?
To enable Lightning Experience in Salesforce:
- Navigate to Setup: Log in to Salesforce and click the gear icon in the upper-right corner, then select “Setup.”
- Lightning Experience Setup: In the Quick Find box, type “Lightning Experience” and select “Lightning Experience Setup.”
- Launch the Migration Assistant: Click “Get Started” in the Lightning Experience Migration Assistant.
- Enable Lightning Experience: Follow the steps in the Migration Assistant to enable Lightning Experience. This will include readiness checks, user setup, and switching users to the new interface.
9. How to create lightning action in Salesforce?
To create a Lightning action in Salesforce:
- Navigate to Setup: Click the gear icon and select “Setup.”
- Object Manager: Go to the Object Manager and select the object where you want to create the action.
- Buttons, Links, and Actions: In the object’s settings, select “Buttons, Links, and Actions.”
- New Action: Click “New Action.” Choose the action type (e.g., Create a Record, Update a Record, Lightning Component).
- Configure the Action: Fill in the details such as action label, name, and description. Specify the fields and layout for the action.
- Save and Add to Page Layout: Save the action and then add it to the appropriate page layout so users can access it.
10. Can we call LWC from a custom button?
Yes, you can call a Lightning Web Component (LWC) from a custom button in Salesforce. One common approach is to create a Quick Action that invokes the LWC. Here’s how:
- Create a LWC: Develop your Lightning Web Component with the desired functionality.
- Create a Lightning Quick Action:
- Navigate to Setup and go to the Object Manager for the object where you want to add the action.
- Select Buttons, Links, and Actions and click New Action.
- Choose Lightning Component as the action type.
- Select your LWC from the dropdown list and configure other details.
- Add Quick Action to the Layout:
- After saving the action, go to the Page Layouts section for the object.
- Edit the layout and add the new action to the Salesforce Mobile and Lightning Experience Actions section.
By following these steps, you can invoke a Lightning Web Component from a custom button in Salesforce, providing a seamless integration of custom functionality.
Are you ready to elevate your Salesforce skills? Dive into our specialized Salesforce Online training in Hyderabad, meticulously designed to provide hands-on experience and real-time knowledge. Our comprehensive, project-based course ensures you gain practical skills with daily notes, engaging projects, and targeted preparation for certifications and interviews, preparing you thoroughly for the dynamic Salesforce ecosystem.
Don’t hesitate to boost your career prospects. Enroll today in our Salesforce course for beginners and benefit from personalized mentorship by seasoned instructors. Whether you’re starting fresh or aiming to refine your Salesforce expertise, our tailored program in Hyderabad is crafted to support your professional growth. Take charge of your career journey with us today.