Question
How to Use a WYSIWYG Editor like TinyMCE, CKEditor, or Quill in LWC for a Managed Package?
I have a use case where I need to integrate a WYSIWYG editor into a managed package using Lightning Web Components (LWC). Specifically, I’m exploring options like TinyMCE, CKEditor, or Quill.
I’ve noticed a managed package on AppExchange that successfully uses CKEditor within an LWC. Is there any way to achieve this integration? Any advice or examples would be helpful!
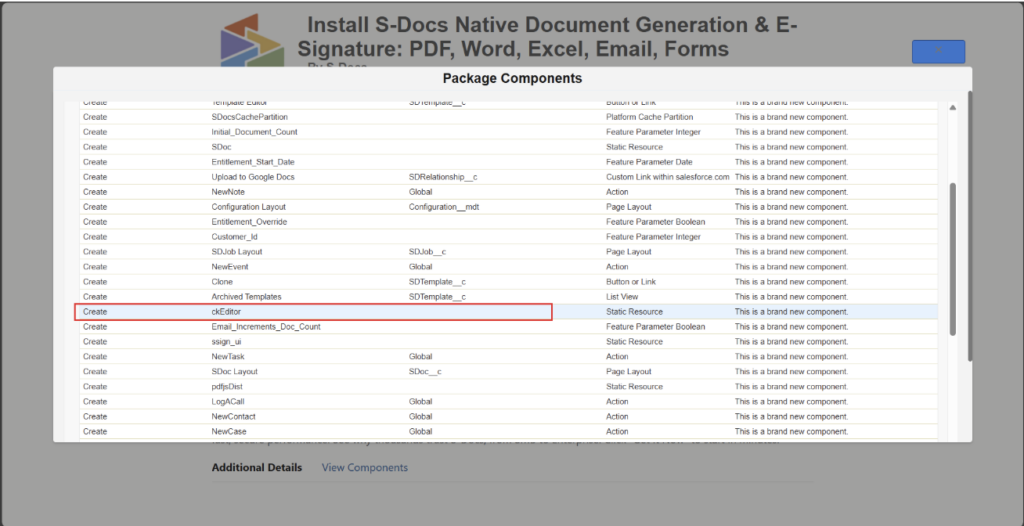
Answer
Yes, it is possible to use WYSIWYG editors like TinyMCE, CKEditor, or Quill in a managed package within Salesforce Lightning Web Components (LWC). However, when integrating third-party WYSIWYG editors into a managed package, certain factors need to be considered, especially in terms of customization and compatibility with Salesforce’s security policies.
- CKEditor: CKEditor is one of the most popular options, and it’s used by many Salesforce developers in managed packages. A managed package using CKEditor can be found on AppExchange. You can include it in an LWC by importing the editor’s JavaScript and configuring it inside the component. However, because you are working within a managed package, you must ensure that CKEditor’s JavaScript libraries are properly bundled and that it does not conflict with Salesforce’s Locker Service, which can restrict access to certain JavaScript functionality.
Example integration in LWC:
import { loadScript, loadStyle } from 'lightning/platformResourceLoader';
import CKEditorLib from '@salesforce/resourceUrl/CKEditor';
export default class MyEditorComponent extends LightningElement {
ckEditorJs;
ckEditorCss;
renderedCallback() {
if (this.ckEditorJs) {
return;
}
this.ckEditorJs = loadScript(this, CKEditorLib + '/ckeditor.js');
this.ckEditorCss = loadStyle(this, CKEditorLib + '/ckeditor.css');
}
handleEditorReady() {
// Initialize the CKEditor after loading
CKEDITOR.replace('editor', {
height: 300,
width: '100%',
});
}
}
Explanation: This code loads CKEditor’s JavaScript and CSS files as static resources using loadScript
and loadStyle
. The editor is initialized in the handleEditorReady
method, where it’s configured with custom height and width. The renderedCallback
method ensures the scripts are loaded only once.
2. TinyMCE: TinyMCE is another robust editor that can be used inside LWC. Similar to CKEditor, TinyMCE must be loaded as a static resource in Salesforce. You can include the editor in your component, but you must ensure that the proper access permissions are set for the static resource and that Salesforce Locker Service does not block critical functionality.
Example integration:
import { loadScript, loadStyle } from 'lightning/platformResourceLoader';
import TinyMCE from '@salesforce/resourceUrl/TinyMCE';
export default class TinyMCEEditor extends LightningElement {
tinymceJs;
tinymceCss;
renderedCallback() {
if (this.tinymceJs) {
return;
}
this.tinymceJs = loadScript(this, TinyMCE + '/tinymce.min.js');
this.tinymceCss = loadStyle(this, TinyMCE + '/tinymce.css');
}
handleEditorReady() {
tinymce.init({
selector: '#editor',
height: 300,
width: '100%',
});
}
}
Explanation: In this code, loadScript
and loadStyle
methods load TinyMCE’s JavaScript and CSS files from a static resource. The handleEditorReady
method initializes the TinyMCE editor, applying configuration options like selector, height, and width for the editor.
3. Quill: Quill is a more lightweight WYSIWYG editor compared to CKEditor and TinyMCE, and it can also be integrated into Salesforce LWC components. Like the other editors, Quill needs to be bundled as a static resource and initialized similarly. Quill is often praised for its simple setup and modern interface.
Example integration:
import { loadScript, loadStyle } from 'lightning/platformResourceLoader';
import QuillLib from '@salesforce/resourceUrl/Quill';
export default class QuillEditor extends LightningElement {
quillJs;
quillCss;
renderedCallback() {
if (this.quillJs) {
return;
}
this.quillJs = loadScript(this, QuillLib + '/quill.min.js');
this.quillCss = loadStyle(this, QuillLib + '/quill.snow.css');
}
handleEditorReady() {
const quill = new Quill('#editor', {
theme: 'snow',
modules: {
toolbar: [['bold', 'italic'], ['link']],
},
placeholder: 'Type here...',
readOnly: false,
theme: 'snow',
});
}
}
Explanation: In this snippet, Quill’s JavaScript and CSS files are loaded using loadScript
and loadStyle
. Once loaded, the Quill editor is initialized in the handleEditorReady
method with a specified toolbar, theme, and other configuration options, including read-only status and placeholder text.
Important Considerations:
- Locker Service: Salesforce’s Locker Service may block certain functionality in the JavaScript libraries of these editors. To avoid compatibility issues, ensure the libraries are compatible with Locker Service. You may need to use
loadScript
andloadStyle
to load the resources properly and ensure the editor works in the secure Locker environment. - Custom Static Resources: Since LWC doesn’t allow external scripts directly, you’ll need to use Salesforce static resources to host the necessary files. This ensures that they are properly encapsulated within your managed package.
- Customization & Maintenance: Each of these editors will have different configuration options and maintenance requirements. You need to ensure that you are maintaining the latest version of the editor and properly bundling the necessary files for deployment.
In conclusion, while TinyMCE, CKEditor, and Quill can all be used in a managed package within LWC, CKEditor is often the easiest option for managed packages due to existing AppExchange integrations. Regardless of the choice, you must ensure the integration complies with Salesforce’s Locker Service policies and that the library is appropriately bundled as a static resource in your Salesforce org.
Our Salesforce training in India provides comprehensive learning with practical exposure to real-world projects and expert guidance. Designed for all skill levels, this course equips you with the expertise to excel in Salesforce CRM. Enroll today and take the first step toward a successful career in Salesforce!
Leave a Reply
You must be logged in to post a comment.