The Apex List Class in Salesforce is a powerful and versatile collection type used to store an ordered collection of elements. Lists in Apex are dynamic, meaning they can grow or shrink in size as elements are added or removed, making them highly flexible for various programming scenarios. This class provides a range of methods to manipulate and interact with the elements, such as adding, removing, and accessing elements by their position. Lists can hold any data type, including primitive types, user-defined objects, sObjects, and collections, making them an essential tool for developers when handling complex data structures.
One of the key advantages of using the Apex List Class is its ability to handle null values and its zero-based indexing, which aligns with many modern programming languages, making it easier for developers to adopt and use. The class supports numerous methods for efficient data manipulation, including add()
, remove()
, get()
, set()
, and more, enabling developers to perform a wide range of operations on the data stored in the list. Additionally, lists can be nested, meaning a list can contain other lists, which is particularly useful for representing hierarchical or multi-dimensional data. With its rich feature set and flexibility, the Apex List Class is a fundamental component in the Salesforce developer’s toolkit, facilitating efficient and effective data management within applications.
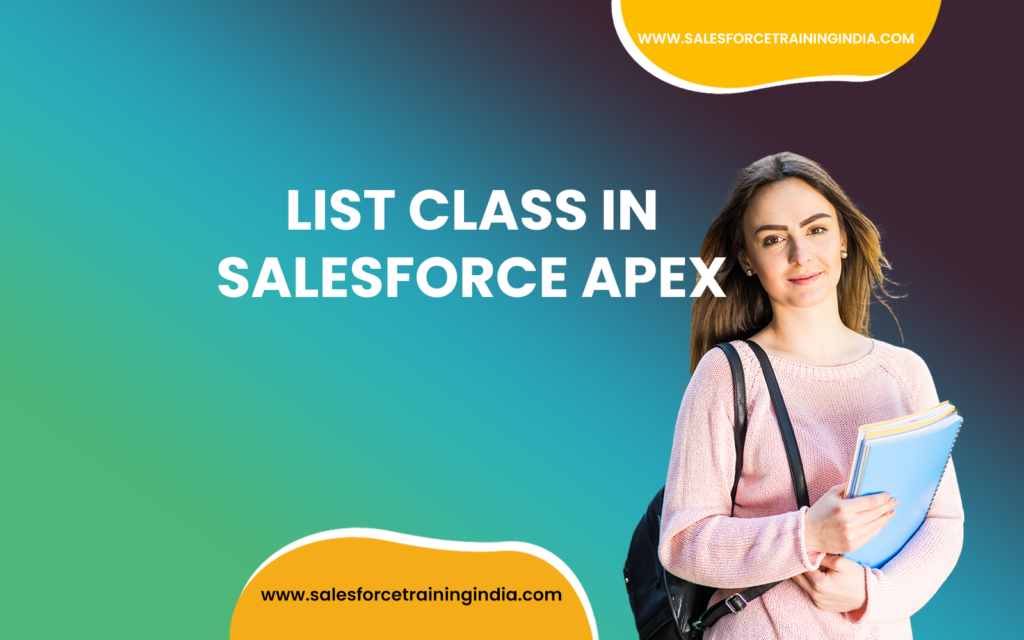
Comparison with Sets and Maps
Here’s a comparison between Apex Lists, Sets, and Maps in a two-column table format:
Feature | Lists | Sets | Maps |
---|---|---|---|
Order | Maintains insertion order | Unordered | Unordered |
Duplicates | Allows duplicates | Does not allow duplicates | Does not allow duplicate keys, but allows duplicate values |
Indexing | Zero-based index for accessing elements | No indexing | Key-based indexing |
Use Case | Suitable for ordered collections with duplicates | Suitable for unique collections | Suitable for key-value pairs |
Methods | add() , get() , set() , remove() , size() | add() , contains() , remove() , size() | put() , get() , remove() , keySet() , values() , size() |
Null Values | Allows null values | Allows null values | Allows null keys and values |
Performance | Efficient for ordered data access and iteration | Efficient for checking unique elements | Efficient for key-based access and retrieval |
Implementation | Dynamic array | Hash-based | Hash-based |
Example | List<Integer> numbers = new List<Integer>(); | Set<String> uniqueNames = new Set<String>(); | Map<String, Integer> scoreMap = new Map<String, Integer>(); |
This table highlights the key differences and use cases of Lists, Sets, and Maps in Apex, helping you choose the appropriate data structure for your specific needs.
When to Use Lists vs. Sets vs. Maps?
Here’s a table summarizing when to use Lists, Sets, and Maps in Apex:
Criteria | Lists | Sets | Maps |
---|---|---|---|
Need for Order | When the order of elements matters | When the order does not matter | When you need to associate keys with values |
Duplicates | When duplicates are allowed | When duplicates must be avoided | When duplicate keys are not allowed |
Access by Index | When you need to access elements by position | Not applicable | When you need to access values by keys |
Unique Elements | Not required | When you need to ensure all elements are unique | Not applicable (keys must be unique) |
Frequent Add/Remove | Suitable for frequent additions/removals at end | Suitable for frequent additions/removals | Suitable for frequent key-value pair additions/removals |
Searching Elements | When position-based search is needed | When existence checking without duplicates is needed | When you need to search values by keys |
Null Handling | Allows null values | Allows null values | Allows null keys and values |
Complex Data Handling | When dealing with ordered, potentially duplicate data | When dealing with a collection of unique elements | When dealing with key-value pairs |
Examples | Handling ordered lists like task lists, queues | Managing sets of unique items like user permissions | Managing associations like user IDs to profiles |
This table helps in choosing the appropriate data structure (Lists, Sets, or Maps) based on specific needs and criteria in Apex programming.
Common Operations with Lists
Here’s the table in a two-column format:
Operation | Example Code |
---|---|
Creating a List | List<String> names = new List<String>(); |
Adding Elements | names.add('Alice'); |
Adding Multiple Elements | names.addAll(new List<String>{'Bob', 'Charlie'}); |
Accessing Elements | String firstName = names.get(0); |
Updating Elements | names.set(1, 'David'); |
Removing Elements | names.remove(0); <br>names.remove('Charlie'); |
Checking List Size | Integer size = names.size(); |
Clearing the List | names.clear(); |
Checking if List is Empty | Boolean isEmpty = names.isEmpty(); |
Iterating Over List | for (String name : names) { System.debug(name); } |
Sorting the List | names.sort(); |
Converting List to Set | Set<String> uniqueNames = new Set<String>(names); |
Cloning the List | List<String> clonedNames = names.clone(); |
Finding Index of Element | Integer index = names.indexOf('Alice'); |
Sublist Extraction | List<String> subList = names.subList(0, 2); |
Contains Check | Boolean containsAlice = names.contains('Alice'); |
Adding Elements at Index | names.add(1, 'Eve'); |
Converting to String | String namesStr = String.join(names, ','); |
Joining Elements | String joinedNames = String.join(names, ';'); |
Removing Duplicates | names = new List<String>(new Set<String>(names)); |
This format should make it easier to read and use the information.
Best Practices and Tips
Here are five best practices and tips for using Lists in Apex:
Best Practice / Tip | Description |
---|---|
1. Initialize Properly | Always initialize your lists to avoid null pointer exceptions. For example, List<String> names = new List<String>(); . |
2. Avoid Hardcoding Indexes | When accessing elements, avoid using hardcoded indexes. Instead, use loops or dynamically determine the index to improve flexibility and maintainability. |
3. Use contains Method for Checks | Before adding elements, use the contains method to check if the list already contains the element to prevent unintended duplicates. |
4. Optimize Performance with Bulk Operations | When adding multiple elements, use the addAll method instead of multiple add calls to reduce CPU time and enhance performance. |
5. Clear Lists Before Reusing | If you need to reuse a list, always clear it using the clear method to avoid unintentional data retention from previous operations. |
These best practices and tips will help ensure efficient and error-free usage of Lists in Apex, leading to better performance and more maintainable code.
The Apex List Class is a dynamic and versatile data structure used to store ordered collections of elements in Salesforce. Lists can grow or shrink as needed, allowing for efficient data management. Key operations include adding, removing, accessing, and iterating over elements. When using Lists, it’s important to follow best practices such as proper initialization, avoiding hardcoded indexes, using the contains
method to prevent duplicates, optimizing performance with bulk operations, and clearing lists before reuse. By adhering to these practices, developers can ensure their code is efficient, maintainable, and less prone to errors.
Frequently Asked Questions (FAQs)
1. What is the List class in Salesforce Apex?
The List class in Salesforce Apex is a collection type that represents an ordered collection of elements. Lists are dynamic in nature, meaning their size can grow or shrink as needed. They are used to store multiple records of any data type, such as primitive data types, collections, sObjects, user-defined types, and built-in Apex types. Lists provide a range of methods for manipulating the data, including adding, removing, and retrieving elements. Understanding the List class is essential for effective data manipulation and management in Apex.
2. How do you declare and initialize a List in Apex?
To declare and initialize a List in Apex, you can use the following syntax:
List<String> names = new List<String>();
names.add('Alice');
names.add('Bob');
In this example, a List of Strings named names
is declared and initialized. Elements ‘Alice’ and ‘Bob’ are then added to the list using the add
method. Lists can be initialized with initial values as well:
List<Integer> numbers = new List<Integer>{1, 2, 3, 4, 5};
This initializes a List of Integers with values 1 through 5.
3. What methods are available in the List class in Apex?
The List class in Apex provides various methods for manipulating lists. Some of the key methods include:
- add(element): Adds an element to the end of the list.
- add(index, element): Inserts an element at the specified index.
- get(index): Returns the element at the specified index.
- set(index, element): Replaces the element at the specified index with a new value.
- remove(index): Removes the element at the specified index.
- size(): Returns the number of elements in the list.
- clear(): Removes all elements from the list. These methods offer flexibility and control over list operations in Apex.
4. How can you iterate over a List in Apex?
To iterate over a List in Apex, you can use several approaches, including for loops and enhanced for loops:
- Standard for loop:
List<String> names = new List<String>{'Alice', 'Bob', 'Charlie'};
for (Integer i = 0; i < names.size(); i++) { System.debug(names.get(i));
}
- Enhanced for loop:
List<String> names = new List<String>{'Alice', 'Bob', 'Charlie'};
for (String name : names) { System.debug(name); }
The enhanced for loop simplifies the code and is more readable when you need to access each element sequentially.
5. How do you handle null values in a List in Apex?
Handling null values in a List in Apex requires careful checks to avoid runtime exceptions. Here are some best practices:
- Check for null before accessing the List:
List<String> names = null;
if (names != null) { // Safe to access list
for (String name : names) {
System.debug(name);
}
}
- Avoid adding null values to the List whenever possible:
List<String> names = new List<String>();
String name = getName(); // Assume this method can return null
if (name != null) { names.add(name); }
By ensuring that null values are appropriately handled, you can maintain the stability and reliability of your Apex code.
Are you eager to master Salesforce and advance your career? Our specialized Salesforce training in India offers a comprehensive, project-based curriculum designed to equip you with real-time knowledge and practical skills. With emphasis on daily notes, hands-on projects, and focused preparation for certification and interviews, our training ensures you are well-prepared for success in the Salesforce ecosystem.
Don’t miss out on this opportunity to elevate your career prospects. Enroll today in our Salesforce course and benefit from personalized mentorship provided by experienced instructors. Whether you’re starting from scratch or aiming to deepen your expertise, our tailored program in Hyderabad is designed to empower your professional journey. Take the next step towards achieving your career goals with us.
Leave a Reply
You must be logged in to post a comment.