Question:
I’m trying to solidify my understanding of the “With Sharing,” “Without Sharing,” and unspecified sharing rules in Salesforce Apex. Below is my current understanding, followed by some scenarios and questions for clarification:
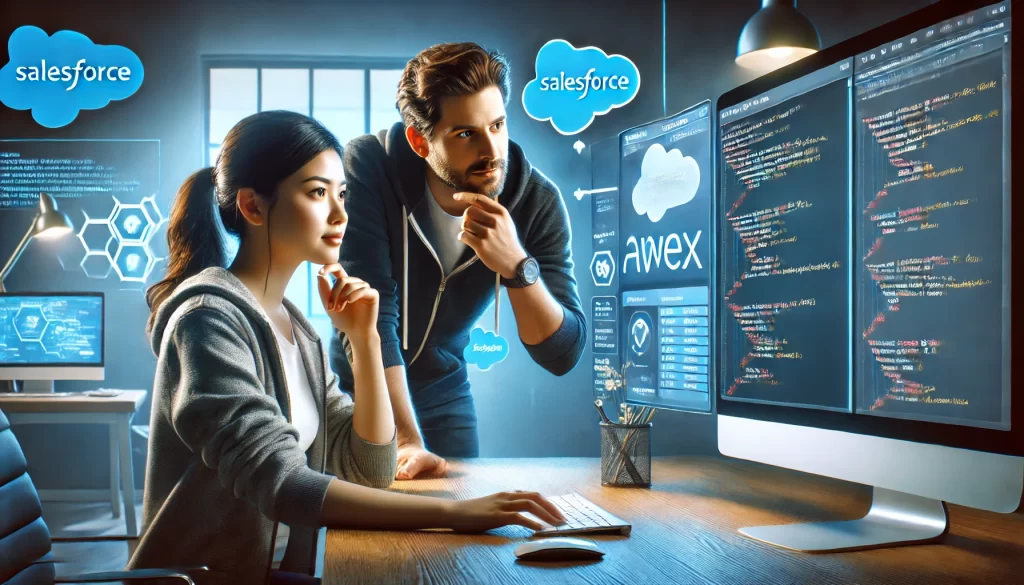
Notes on Sharing Rules:
- With Sharing:
- Implies “with security settings enforced”, specifically respecting OWDs and Sharing Rules.
- Question: Does this also apply to Object-Level Security (OLS) and Field-Level Security (FLS)?
- If a class is declared with sharing, the sharing settings apply to:
- All code in the class (including initialization, constructors, and methods).
- Exception: Inner classes do not inherit sharing settings from their outer class.
- Unspecified Sharing:
- Classes not explicitly declared with or without sharing run in system mode (without sharing).
- However, if a “with sharing” class calls such a class, it executes in “with sharing” mode by default.
- Inheritance of Sharing Rules:
- If class B extends class A, B inherits A’s sharing mode.
- Inner classes of B do not inherit B’s sharing settings.
- Method Invocation Across Classes:
- If a with sharing class calls a without sharing class, the called methods execute in the mode of their defining class (in this case, without sharing).
Scenarios for Validation:
I have three classes:
public with sharing class A {}
public without sharing class B {}
public class C {} // Unspecified sharing
Based on the above, I have the following scenarios:
class B extends class A
:- Code in class B executes in “with sharing” mode (inherits parent class A’s mode).
class B calls class A
:- Called code in class A executes in “with sharing” mode (mode of the defining class).
class C calls class A
:- Called code in class A executes in “with sharing” mode (mode of the defining class).
class C extends class A
:- Code in class C executes in “with sharing” mode (inherits parent class A’s mode).
class A calls class C
:- Code in class C executes in system mode (since class C is unspecified and runs without sharing).
class A extends class C
:- Code in class A executes in “without sharing” mode (inherits parent class C’s mode).
- Question: Is this understanding correct?
Additional Questions:
- What are common use cases where “with sharing” and “without sharing” rules are explicitly applied?
- How do these sharing settings apply in the context of Visualforce Controller Classes and Controller Extensions?
Keywords: Apex, sharing rules, with sharing, without sharing, unspecified sharing, inheritance, Visualforce controllers, Salesforce Apex security.
Answer
1. With Sharing
When a class is declared with the with sharing
keyword, it enforces the sharing rules of the current user. This includes organization-wide defaults (OWD) and sharing rules but does not automatically enforce object-level security (OLS) or field-level security (FLS). Developers must handle OLS and FLS programmatically.
public with sharing class ClassA {
public void methodA() {
// This query respects sharing rules
List<Account> accounts = [SELECT Id FROM Account];
}
}
2. Without Sharing
A class declared with the without sharing
keyword explicitly ignores sharing rules. It executes in system mode, bypassing the user’s sharing rules. This is useful for system processes or operations that require elevated access.
public without sharing class ClassB {
public void methodB() {
// This query ignores sharing rules
List<Account> accounts = [SELECT Id FROM Account];
}
}
3. Unspecified Sharing (Default Behavior)
If a class does not explicitly declare with sharing
or without sharing
, it executes in system mode (ignoring sharing rules) except in certain contexts, like Execute Anonymous or Chatter, where it behaves as with sharing
. When such a class is invoked by another class with sharing, it inherits the caller’s sharing mode.
public class ClassC {
public void methodC() {
// Executes in system mode unless called from a `with sharing` context
List<Account> accounts = [SELECT Id FROM Account];
}
}
Behavior in Scenarios
Scenario 1:
If Class B extends Class A (with sharing
), Class B inherits the sharing settings of Class A. This means all methods in Class B execute in with sharing
mode.
public with sharing class ClassA {}
public without sharing class ClassB extends ClassA {} // ClassB now enforces sharing rules
Scenario 2:
If Class B calls a method in Class A (with sharing
), the method executes in with sharing
mode, respecting sharing rules.
ClassA a = new ClassA();
a.methodA(); // Executes in `with sharing` mode
Scenario 3:
If Class C (unspecified sharing) calls Class A (with sharing
), the method in Class A executes in with sharing
mode, inheriting its own declaration.
ClassA a = new ClassA();
ClassC c = new ClassC();
c.someMethod(); // Method in ClassA respects its `with sharing` mode
Scenario 4:
If Class A (with sharing
) calls Class C (unspecified sharing), Class C executes in system mode unless it is called from an environment like Execute Anonymous, where it behaves as with sharing
.
ClassA a = new ClassA();
a.callClassC(); // ClassC executes in system mode
Scenario 5:
If Class A extends Class C (unspecified sharing), Class A’s behavior depends on its declaration. If Class A is declared with sharing
, it overrides Class C’s default behavior.
public class ClassC {}
public with sharing class ClassA extends ClassC {} // ClassA enforces sharing rules
Use Cases for Sharing Settings
- Use
with sharing
for user-facing operations, such as Visualforce pages or Lightning components, where data visibility must adhere to the user’s permissions. - Use
without sharing
for system-level processes, like logging, data cleanup, or administrative tasks, where bypassing sharing rules is necessary.
In Visualforce Controller Classes and Extensions, sharing settings are crucial. Controllers that directly fetch or update user data should generally use with sharing
to ensure adherence to user permissions. However, controller extensions designed for administrative functions might use without sharing
for broader access.
These rules provide a robust mechanism to control data access in Salesforce while balancing flexibility and security.
Summing Up
In Salesforce Apex, sharing settings dictate how data access is controlled during code execution.
- With Sharing enforces user-specific sharing rules, such as organization-wide defaults and sharing rules. It is ideal for user-facing operations to respect data visibility.
- Without Sharing bypasses sharing rules, allowing system-level processes to execute without restriction.
- Unspecified Sharing defaults to system mode but inherits the sharing mode of the caller when invoked from a
with sharing
context.
Sharing settings do not automatically enforce object-level or field-level security, requiring explicit handling by the developer. When classes interact, the sharing mode depends on their declarations and context, ensuring flexible yet secure access to data. For Visualforce controllers and extensions, using with sharing
is common unless broader access is necessary for administrative tasks.
Our Salesforce training in Bangalore offers an in-depth dive into the Salesforce platform, arming you with the essential skills to thrive in the competitive CRM industry. The program covers key areas like Salesforce Admin, Developer, and AI, blending theoretical knowledge with hands-on practical experience. Participate in live projects and assignments to gain real-world expertise and confidently address business challenges using Salesforce solutions. Our experienced trainers ensure you acquire the technical know-how and industry insight necessary to excel in the Salesforce ecosystem.
Beyond technical skills, our Salesforce training in Bangalore provides personalized mentorship, certification exam preparation, and interview coaching to help you stand out in the job market. You will have access to a wealth of study resources, real-world project exposure, and continuous support throughout your learning journey. Upon completion, you’ll be fully prepared for certification exams and equipped with the problem-solving abilities employers seek. Begin your Salesforce journey with us and unlock a world of career opportunities!